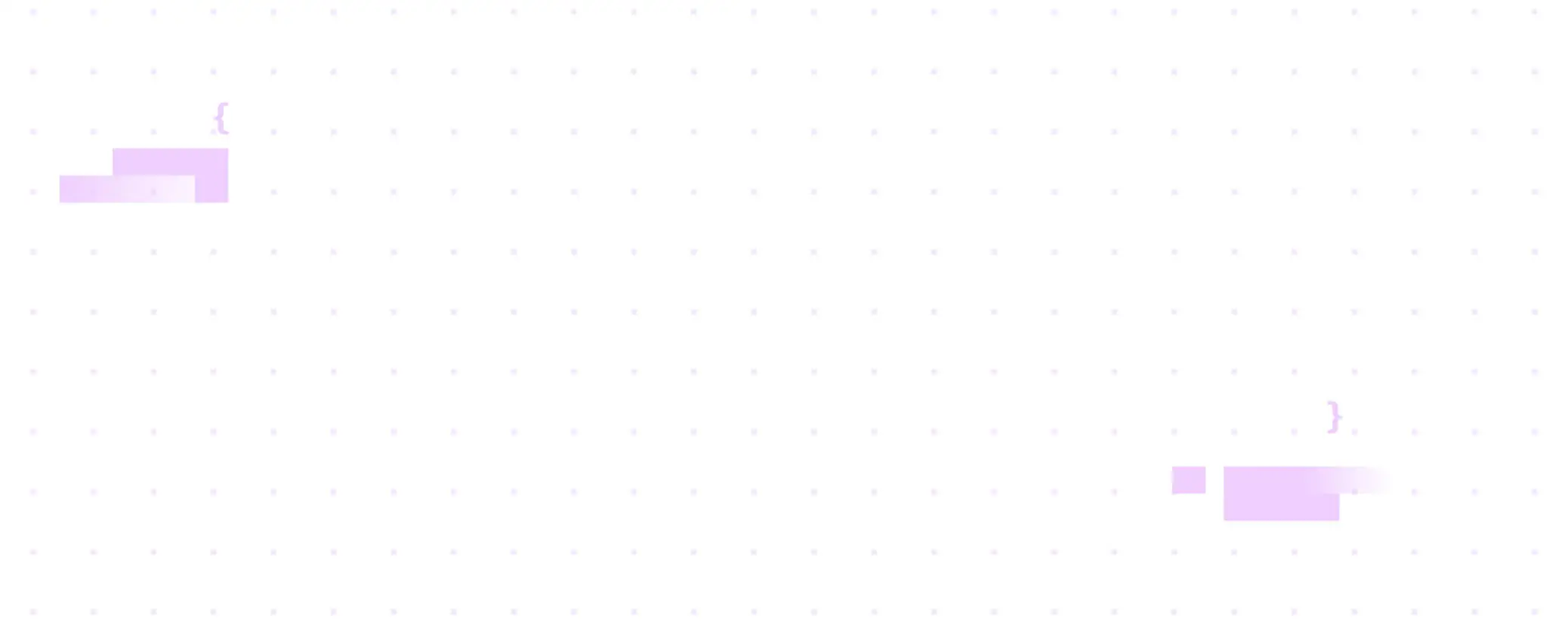
Modernize and maintain legacy code faster
Generate comprehensive documentation for any programming language – from COBOL to modern
Legacy projects are high-risk and constantly behind schedule when the codebase is a black box
Automatically generate code documentation
Backfill missing documentation
Turn your spaghetti code into clear explanations with examples that enable your engineers to quickly understand the most complex codebases
Generate system and
code diagrams
Give product managers, architects, and engineers the information they need to understand every level of your system, from conceptual to code implementation
Never lose the context behind your legacy code
Auto-sync keeps documentation up to date as part of the developer’s CI process
Explore Swimm COBOL documentation
Processing Account Creation (CREACC)
The CREACC program is responsible for creating new bank accounts. It starts by setting up necessary fields, validating customer information, and then proceeds to count and validate customer accounts. The program ensures that only valid account types are processed and handles any errors that may occur during the process. Finally, it enqueues a named counter, finds the next available account number, and writes the new account details to the DB2
database.
The flow starts with setting up fields and validating customer information. If the customer is valid, it counts and validates the customer’s accounts. It then ensures the account type is valid, enqueues a named counter, finds the next available account number, and writes the new account details to the DB2
database.
Where is this program used?
This program is used once, in a flow starting from BNK1CAC
as represented in the following diagram:
Here is a high level diagram of the program:
Setups
Moving SORTCODE to REQUIRED-SORT-CODE
First, the SORTCODE
is moved to REQUIRED-SORT-CODE
and REQUIRED-SORT-CODE2
. This step ensures that the sort code is correctly set up for further processing.
MOVE SORTCODE TO
REQUIRED-SORT-CODE
REQUIRED-SORT-CODE2.
Moving ZERO to ACCOUNT-NUMBER
Next, the ACCOUNT-NUMBER
is initialized to zero. This step prepares the account number field for subsequent operations.
MOVE ZERO TO ACCOUNT-NUMBER.
Initializing INQCUST-COMMAREA
Then, the INQCUST-COMMAREA
is initialized. This step sets up the communication area for the INQCUST
program call.
INITIALIZE INQCUST-COMMAREA
Moving COMM-CUSTNO
to INQCUST-CUSTNO
Moving to the next step, the COMM-CUSTNO
from DFHCOMMAREA
is moved to INQCUST-CUSTNO
. This step transfers the customer number to the communication area for validation.
MOVE COMM-CUSTNO IN DFHCOMMAREA TO INQCUST-CUSTNO.
Calling INQCUST Program
Finally, the INQCUST
program is called using the EXEC CICS LINK
command. This step validates the existence of the customer by linking to the INQCUST
program.
More about INQCUST: Get Customer Record (INQCUST)
EXEC CICS LINK PROGRAM('INQCUST ')
COMMAREA(INQCUST-COMMAREA)
RESP(WS-CICS-RESP)
END-EXEC.
Customer validation
Call to INQCUST
First, the program makes a call to the INQCUST
program to retrieve customer information. This is done using the EXEC CICS LINK
command, which links to the INQCUST
program and passes the communication area INQCUST-COMMAREA
.
More about INQCUST: Get Customer Record (INQCUST)
EXEC CICS LINK PROGRAM('INQCUST ')
COMMAREA(INQCUST-COMMAREA)
RESP(WS-CICS-RESP)
END-EXEC.
Check Response
Next, the program checks the response from the INQCUST
call. It verifies if the response code EIBRESP
is normal and if the INQCUST-INQ-SUCCESS
flag is set to ‘Y’. If either of these conditions is not met, it indicates a failure in retrieving customer information.
IF EIBRESP IS NOT EQUAL TO DFHRESP(NORMAL)
OR INQCUST-INQ-SUCCESS IS NOT EQUAL TO 'Y'
Handle Failure
If the response check fails, the program sets the COMM-SUCCESS
flag to ‘N’ and the COMM-FAIL-CODE
to ‘1’ in the DFHCOMMAREA
. It then performs the GET-ME-OUT-OF-HERE
section to handle the failure and exit.
MOVE 'N' TO COMM-SUCCESS IN DFHCOMMAREA
MOVE '1' TO COMM-FAIL-CODE IN DFHCOMMAREA
PERFORM GET-ME-OUT-OF-HERE
END-IF
Count Customer Accounts
If the response check is successful, the program proceeds to the CUSTOMER-ACCOUNT-COUNT
section. This section sets the number of accounts to 20 and moves the customer number from DFHCOMMAREA
to INQACCCU-COMMAREA
. It then makes a call to the INQACCCU
program to retrieve the account details.
CUSTOMER-ACCOUNT-COUNT SECTION.
CAC010.
MOVE 20 TO NUMBER-OF-ACCOUNTS IN INQACCCU-COMMAREA.
MOVE COMM-CUSTNO IN DFHCOMMAREA
TO CUSTOMER-NUMBER IN INQACCCU-COMMAREA.
SET COMM-PCB-POINTER TO NULL
EXEC CICS LINK PROGRAM('INQACCCU')
COMMAREA(INQACCCU-COMMAREA)
RESP(WS-CICS-RESP)
SYNCONRETURN
END-EXEC.
CAC999.
EXIT.
Interim Summary
So far, we saw how the program initializes various fields and makes a call to the INQCUST
program to validate the customer. If the validation is successful, it proceeds to count the customer accounts by calling the INQACCCU
program. Now, we will focus on how the program retrieves customer account information and handles any errors that may occur during this process.
Account information fetching and validation
Retrieve Customer Account Information
First, the program performs the CUSTOMER-ACCOUNT-COUNT
paragraph to retrieve customer account information.
PERFORM CUSTOMER-ACCOUNT-COUNT.
Check Response Status
Next, the program checks if the response status WS-CICS-RESP
is not equal to DFHRESP(NORMAL)
. If the response is not normal, it displays an error message, sets the communication success flag to ‘N’, sets the communication failure code to ‘9’, and then performs the GET-ME-OUT-OF-HERE
paragraph to exit the process.
IF WS-CICS-RESP IS NOT EQUAL TO DFHRESP(NORMAL)
DISPLAY 'Error counting accounts'
MOVE 'N' to COMM-SUCCESS IN DFHCOMMAREA
MOVE 'N' to COMM-SUCCESS IN INQACCCU-COMMAREA
MOVE '9' TO COMM-FAIL-CODE IN DFHCOMMAREA
PERFORM GET-ME-OUT-OF-HERE
END-IF
Check Communication Success
Then, the program checks if the communication success flag COMM-SUCCESS
in INQACCCU-COMMAREA
is ‘N’. If it is, it displays an error message, sets the communication success flag to ‘N’, sets the communication failure code to ‘9’, and then performs the GET-ME-OUT-OF-HERE
paragraph to exit the process.
IF COMM-SUCCESS IN INQACCCU-COMMAREA = 'N'
DISPLAY 'Error counting accounts'
MOVE 'N' to COMM-SUCCESS IN DFHCOMMAREA
MOVE '9' TO COMM-FAIL-CODE IN DFHCOMMAREA
PERFORM GET-ME-OUT-OF-HERE
END-IF
Account type validation
Checking Number of Accounts
First, the code checks if the number of accounts in INQACCCU-COMMAREA
is greater than 9. If true, it sets COMM-SUCCESS
to ‘N’ and COMM-FAIL-CODE
to ‘8’, then performs GET-ME-OUT-OF-HERE
to exit.
IF NUMBER-OF-ACCOUNTS IN INQACCCU-COMMAREA > 9
MOVE 'N' TO COMM-SUCCESS IN DFHCOMMAREA
MOVE '8' TO COMM-FAIL-CODE IN DFHCOMMAREA
PERFORM GET-ME-OUT-OF-HERE
END-IF
Validating Account Type
Next, the code performs ACCOUNT-TYPE-CHECK
to validate the type of account. This ensures that only valid account types like ISA, MORTGAGE, SAVING, CURRENT, and LOAN are accepted.
* Validate the type of account e.g. MORTGAGE etc.
*
PERFORM ACCOUNT-TYPE-CHECK
ACCOUNT-TYPE-CHECK
The ACCOUNT-TYPE-CHECK
section evaluates the account type in DFHCOMMAREA
. If the account type matches one of the valid types, it sets COMM-SUCCESS
to ‘Y’. Otherwise, it sets COMM-SUCCESS
to ‘N’ and COMM-FAIL-CODE
to ‘A’.
ACCOUNT-TYPE-CHECK SECTION.
ATC010.
*
* Validate that only ISA, MORTGAGE, SAVING, CURRENT and LOAN
* are the only account types available.
*
EVALUATE TRUE
WHEN COMM-ACC-TYPE IN DFHCOMMAREA(1:3) = 'ISA'
WHEN COMM-ACC-TYPE IN DFHCOMMAREA(1:8) = 'MORTGAGE'
WHEN COMM-ACC-TYPE IN DFHCOMMAREA(1:6) = 'SAVING'
WHEN COMM-ACC-TYPE IN DFHCOMMAREA(1:7) = 'CURRENT'
WHEN COMM-ACC-TYPE IN DFHCOMMAREA(1:4) = 'LOAN'
MOVE 'Y' TO COMM-SUCCESS OF DFHCOMMAREA
WHEN OTHER
MOVE 'N' TO COMM-SUCCESS OF DFHCOMMAREA
MOVE 'A' TO COMM-FAIL-CODE IN DFHCOMMAREA
END-EVALUATE.
ATC999.
EXIT.
Checking Validation Success
Then, the code checks if COMM-SUCCESS
is ‘N’. If true, it performs GET-ME-OUT-OF-HERE
to exit.
IF COMM-SUCCESS OF DFHCOMMAREA = 'N'
PERFORM GET-ME-OUT-OF-HERE
END-IF
ENQ-NAMED-COUNTER
The ENQ-NAMED-COUNTER
section enqueues a named counter using the SORTCODE
. If the response is not normal, it sets COMM-SUCCESS
to ‘N’ and COMM-FAIL-CODE
to ‘3’, then performs GET-ME-OUT-OF-HERE
to exit.
ENQ-NAMED-COUNTER SECTION.
ENC010.
MOVE SORTCODE TO NCS-ACC-NO-TEST-SORT.
EXEC CICS ENQ
RESOURCE(NCS-ACC-NO-NAME)
LENGTH(16)
RESP(WS-CICS-RESP)
RESP2(WS-CICS-RESP2)
END-EXEC.
IF WS-CICS-RESP NOT = DFHRESP(NORMAL)
MOVE 'N' TO COMM-SUCCESS IN DFHCOMMAREA
MOVE '3' TO COMM-FAIL-CODE IN DFHCOMMAREA
PERFORM GET-ME-OUT-OF-HERE
END-IF.
ENC999.
EXIT.
Account processing and outputs
Enqueue Named Counter
First, we enqueue the named counter for the account. This ensures that the account number generation is synchronized and unique.
PERFORM ENQ-NAMED-COUNTER.
Find Next Account
Next, we perform the FIND-NEXT-ACCOUNT
operation to retrieve the next available account number from the account control table.
PERFORM FIND-NEXT-ACCOUNT.
FIND-NEXT-ACCOUNT
The FIND-NEXT-ACCOUNT
section increments the account number and retrieves the current control values from the database. If the SQL operation fails, it handles the error by logging and abending the transaction.
FIND-NEXT-ACCOUNT SECTION.
FNA010.
MOVE 1 TO NCS-ACC-NO-INC.
INITIALIZE OUTPUT-DATA.
<span"> * <<sortcode>>-ACCOUNT-LAST
MOVE SPACES TO HV-CONTROL-NAME
MOVE ZERO TO HV-CONTROL-VALUE-NUM
MOVE SPACES TO HV-CONTROL-VALUE-STR
STRING REQUIRED-SORT-CODE DELIMITED BY SIZE
'-' DELIMITED BY SIZE
'ACCOUNT-LAST' DELIMITED BY SIZE
INTO HV-CONTROL-NAME
EXEC SQL
SELECT CONTROL_NAME,
CONTROL_VALUE_NUM,
CONTROL_VALUE_STR
INTO :HV-CONTROL-NAME,
:HV-CONTROL-VALUE-NUM,
Write Account to DB2
Then, we perform the WRITE-ACCOUNT-DB2
operation to insert the new account details into the DB2
database.
PERFORM WRITE-ACCOUNT-DB2
WRITE-ACCOUNT-DB2
The WRITE-ACCOUNT-DB2
section initializes the account row, sets the account details, calculates the next statement date, and inserts the account into the DB2
database. If the insert fails, it handles the error by logging and abending the transaction.
WRITE-ACCOUNT-DB2 SECTION.
WAD010.
INITIALIZE HOST-ACCOUNT-ROW.
MOVE 'ACCT' TO HV-ACCOUNT-EYECATCHER.
MOVE COMM-CUSTNO IN DFHCOMMAREA TO HV-ACCOUNT-CUST-NO.
MOVE SORTCODE TO HV-ACCOUNT-SORTCODE.
MOVE NCS-ACC-NO-VALUE TO NCS-ACC-NO-DISP.
MOVE NCS-ACC-NO-DISP(9:8) TO HV-ACCOUNT-ACC-NO.
MOVE COMM-ACC-TYPE IN DFHCOMMAREA TO HV-ACCOUNT-ACC-TYPE.
MOVE COMM-INT-RT TO HV-ACCOUNT-INT-RATE.
MOVE COMM-OVERDR-LIM TO HV-ACCOUNT-OVERDRAFT-LIM.
MOVE COMM-AVAIL-BAL IN DFHCOMMAREA TO HV-ACCOUNT-AVAIL-BAL.
MOVE COMM-ACT-BAL TO HV-ACCOUNT-ACTUAL-BAL.
PERFORM CALCULATE-DATES.
*
* Convert gregorian date (YYYYMMDD) to an integer
*
This is an auto-generated document by Swimm 🌊 and has not yet been verified by a human
How it works
Don’t let legacy code hold
your projects back
“Swimm is a tool that we don’t know how we lived without. Easily accessible information improved productivity and made transferring knowledge between engineers frictionless.”